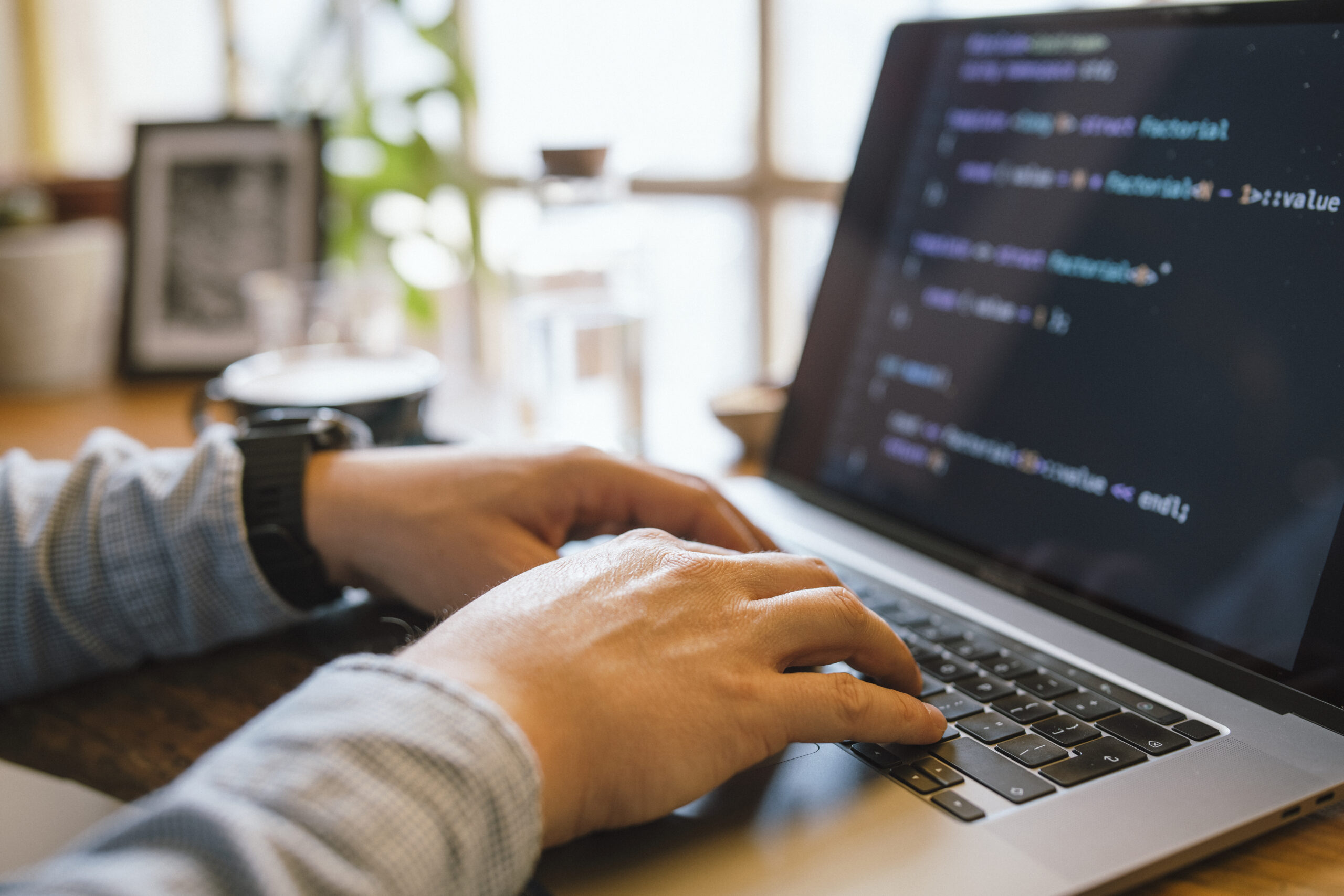
Debugging is One of the more important — nevertheless normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much fixing broken code; it’s about comprehension how and why points go Erroneous, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can save several hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
On the list of fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. While crafting code is just one Section of advancement, understanding how to interact with it correctly through execution is equally important. Fashionable growth environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface of what these equipment can do.
Acquire, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, action by means of code line by line, and even modify code on the fly. When applied appropriately, they Permit you to observe exactly how your code behaves through execution, which happens to be priceless for tracking down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for entrance-close developers. They enable you to inspect the DOM, monitor network requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, sources, and network tabs can transform aggravating UI issues into manageable responsibilities.
For backend or technique-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about operating procedures and memory management. Finding out these applications may have a steeper Studying curve but pays off when debugging functionality concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Handle devices like Git to understand code heritage, obtain the precise moment bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your tools indicates going past default options and shortcuts — it’s about establishing an intimate expertise in your development atmosphere to ensure that when concerns come up, you’re not dropped at nighttime. The greater you know your tools, the greater time you could expend resolving the particular problem instead of fumbling via the process.
Reproduce the Problem
Probably the most crucial — and often disregarded — actions in efficient debugging is reproducing the problem. Before leaping to the code or producing guesses, developers want to create a dependable natural environment or situation where the bug reliably seems. Without reproducibility, correcting a bug turns into a sport of chance, generally resulting in wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask issues like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater element you've, the a lot easier it gets to isolate the exact circumstances less than which the bug happens.
After you’ve gathered adequate information, try and recreate the issue in your neighborhood environment. This may suggest inputting a similar knowledge, simulating identical consumer interactions, or mimicking process states. If the issue appears intermittently, contemplate crafting automated assessments that replicate the sting instances or condition transitions involved. These checks not just support expose the problem but in addition avoid regressions Down the road.
Occasionally, The difficulty might be setting-unique — it might take place only on selected operating methods, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, along with a methodical strategy. But as you can consistently recreate the bug, you're presently halfway to fixing it. Having a reproducible situation, You may use your debugging applications extra effectively, test possible fixes safely, and communicate more Obviously using your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages tend to be the most respected clues a developer has when anything goes Improper. As opposed to seeing them as discouraging interruptions, builders must discover to treat error messages as immediate communications with the technique. They frequently tell you what precisely took place, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in full. Quite a few developers, especially when underneath time stress, look at the primary line and promptly commence making assumptions. But further in the mistake stack or logs might lie the legitimate root bring about. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or purpose triggered it? These questions can information your investigation and point you toward the liable code.
It’s also beneficial to be familiar with the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java normally stick to predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check connected log entries, enter values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These generally precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint difficulties a lot quicker, reduce debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective applications in a developer’s debugging toolkit. When used successfully, it provides real-time insights into how an application behaves, helping you understand what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with knowing what to log and at what amount. Typical logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic information during improvement, INFO for general situations (like successful get started-ups), Alert for likely concerns that don’t break the appliance, ERROR for genuine troubles, and FATAL when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant information. Too much logging can obscure vital messages and decelerate your method. Deal with critical activities, point out improvements, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to keep track of how variables evolve, what disorders are fulfilled, and what branches of logic are executed—all without halting the program. They’re especially useful in output environments in which stepping as a result of code isn’t achievable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, you may reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your code.
Feel Just like a Detective
Debugging is not simply a technological task—it's a type of investigation. To properly detect and fix bugs, builders will have to approach the process like a detective fixing a thriller. This frame of mind can help stop working complex troubles into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you could without having jumping to conclusions. Use logs, check conditions, and person stories to piece jointly a clear image of what’s happening.
Next, kind hypotheses. Request oneself: What could possibly be creating this behavior? Have any changes a short while ago been designed on the codebase? Has this situation transpired ahead of below similar instances? The target is usually to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Make an effort to recreate the issue inside of a managed atmosphere. If you suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code queries and Enable the final results guide you nearer to the truth.
Pay near notice to modest particulars. Bugs normally conceal in the minimum expected spots—like a lacking semicolon, an off-by-one mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes may perhaps conceal the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming difficulties and assist Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering concealed problems in intricate devices.
Write Exams
Composing assessments is among the simplest ways to boost your debugging competencies and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a security Web that offers you self-confidence when producing alterations on your codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint particularly where by and when a dilemma occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular bit of logic is Performing as predicted. Each time a check fails, you instantly know exactly where to look, significantly reducing some time expended debugging. Unit tests are Specially beneficial for catching regression bugs—concerns that reappear following Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These help make sure a variety of elements of your software get the job done collectively smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous components or expert services interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To test a element correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. Once the take a look at fails consistently, you'll be able to deal with fixing the bug and observe your take a look at pass when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you capture additional bugs, faster and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the trouble—watching your display screen for hrs, hoping Alternative soon after Option. But One of the more underrated debugging tools is simply stepping absent. Taking breaks helps you reset your mind, reduce aggravation, and often see the issue from a new standpoint.
If you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain will become considerably less productive at difficulty-solving. A brief wander, a espresso split, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report finding the basis of a difficulty after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically during for a longer period debugging periods. Sitting in front of a display screen, mentally caught, is not only unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
Should you’re stuck, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may experience counterintuitive, Specifically less than restricted deadlines, but it really really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It provides your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is much more than simply A short lived setback—It is a chance to improve to be a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you anything precious for those who make an effort to reflect and assess what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code assessments, or logging? The responses frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you realized. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be Primarily highly effective. No matter whether it’s through a Slack information, a short generate-up, or a quick understanding-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical elements of your advancement journey. After all, several of the very best builders are not those who write excellent code, but read more those who continually learn from their problems.
In the end, Each and every bug you take care of adds a completely new layer for your ability established. So subsequent time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — however the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.